Two-level operators
Reference: Nina S. T. Hirata, "Multilevel Training of Binary Morphological Operators" at IEEE Transactions on Pattern Analysis and Machine Intelligence, 2009.
Two level operators are an approach to combine several image transforms that use different (and relatively small) into a single (and better) image transform. It works by building a new pattern using the output of the combined image transforms. The figure below illustrates this process when combining three image transforms.
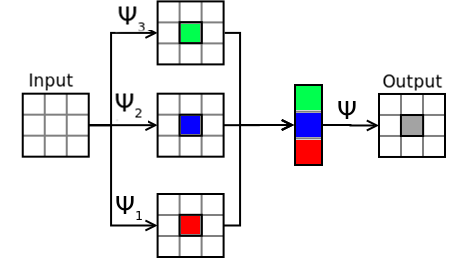
Designing these combinations of image transforms requires the determination of how many image transforms to combine and how to determine the parameters of each one. A simple yet effective approach is to take randomly subsets of moderate size of a large neighborhood. The code below exemplifies this approach in the jung dataset. Although results are better than the basic approach, it is still worse than using more sofisticated methods.
Two-level is implemented as a feature extractor called CombinationPattern
. It
receives operators as arguments and computes the colored feature vector in the
figure above.
# file docs/examples/methods/two-level.py
from trios.classifiers import SKClassifier
from sklearn.tree import DecisionTreeClassifier
from trios.feature_extractors import RAWFeatureExtractor, CombinationPattern
import trios
import numpy as np
import trios.shortcuts.persistence as p
import trios.shortcuts.window as w
if __name__ == '__main__':
np.random.seed(10) # set this to select the same window everytime
images = trios.Imageset.read('../jung-images/level1.set')
images2 = trios.Imageset.read('../jung-images/level2.set')
test = trios.Imageset.read('../jung-images/test.set')
domain = np.ones((9, 7), np.uint8)
windows = [w.random_win(domain, 40, True) for i in range(5)]
ops = []
for i in range(5):
op = trios.WOperator(windows[i], SKClassifier(DecisionTreeClassifier()), RAWFeatureExtractor)
print('Training...', i)
op.train(images)
ops.append(op)
comb = CombinationPattern(*ops)
wop2 = trios.WOperator(comb.window, SKClassifier(DecisionTreeClassifier()), comb)
print('Training 2nd level')
wop2.train(images2)
print('Error', wop2.eval(test))
Training... 0 Training... 1 Training... 2 Training... 3 Training... 4 Training 2nd level Error 0.007666954366731778
An approach that has also shown to be effective is to use basic shapes such as crosses, lines and rectangles in the individual image transforms. This page shows some windows used when processing music documents.
The NILC method is an evolution of this random combination method and should achieve much better results.